Prep Questions¶
PREP QUESTIONS YOU MUST SUBMIT:
- Calculate The Bill
- Cipher
- Baseball Stats
Submit these to owl before the deadline. Put them in separate functions in a single .py file.
EXAM DAY AND TIME:
DEC 19th, 2017:
7pm-10pm
EXAM LOCATIONS:
HSB 13
HSB 14
MC 235
Details on which room you are in will be released shortly.
DURING THE EXAM...
You must use a lab computer and you must not talk to one another. You may use the google, or any other website to complete the questions (however, you’re not allowed to upload solutions at an early date, and then download/copy them during the practical). Be warned that the lab computers will monitored during this time.
The lab computers use Microsoft Windows, so I recommend visiting these labs at an early date to familiarize yourself with the environment if you’re not already comfortable with Windows. You are only allowed to use the ipython interpreter and Notepad++ (no IDEs are allowed).
You are not allowed to go to the TAs or me for help with these questions!
Comments are an important part of any code!
- Google is going to be your best friend!
- Don’t know how to stop a loop? GOOGLE IT!
- Don’t know how to add quotes within a string? GOOGLE IT!
- etc.
1. Multiples of 3 and/or 5¶
If someone were to list all the natural numbers below 10 that are multiples of 3 or 5 we get 3,5,6, and also 9. The sum of these values is 23 (3 + 5 + 6 + 9 = 23).
If we are to do multiples of 3 or 5 below 20, we get 3, 5, 6, 9, 10, 12, 15, and 18. The sum of these is 78.
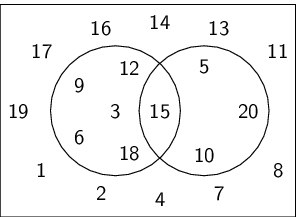
Write a program to find the sum of all the multipls of 3 or 5 below 1000.
NOTES:
- Be sure to test your code on the above examples
2. Special Pythagorean Triplet¶
A Pythagorean truplet is a set of three natural numbers (just think positive integers) where:
a < b < c, for which: a^2 + b^2 = c^2.
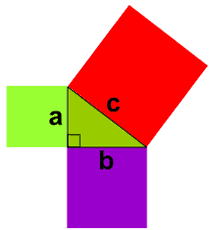
Example:
a = 3, b = 4, c = 5
3^2 + 4^2 = 9 + 16 = 25 = 5^2
There exists exactly one Pythagorean triplet for which a + b + c = 1000. Write a program to find a b and c.
NOTES:
- If you do this poorly it might take a long time to find the solution
- This is totally important because you might have to do this one day:
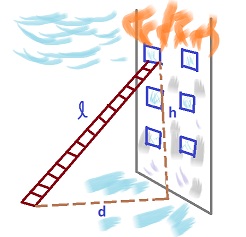
3. Calculate The Bill¶
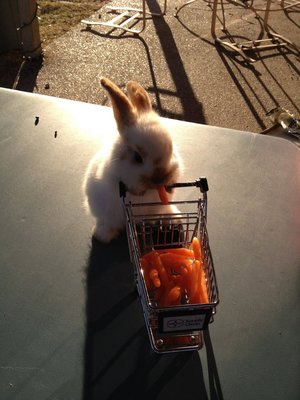
Write a program to calculate this bunny’s bill.
- The program will continuously prompt the user to enter the name of the item purchased, then prompt for the price of the item, and lastly ask for the quantity. This process will repeat until the user types “CHK” in capital letters.
- After “CHK” is typed, the program will ask for the tax rate.
- The program will display a formatted table like the example displayed below.
NOTES:
- The program should work for an arbitrary large number of items
- Assume he doesn’t only buy carrots
Your program must show exactly 2 decimal places when printing the bill
Your program must round properly
- Formatting is important!
- However, don’t worry about having the columns aligned up perfectly based on digit placement (ex, tax row within the totals column)
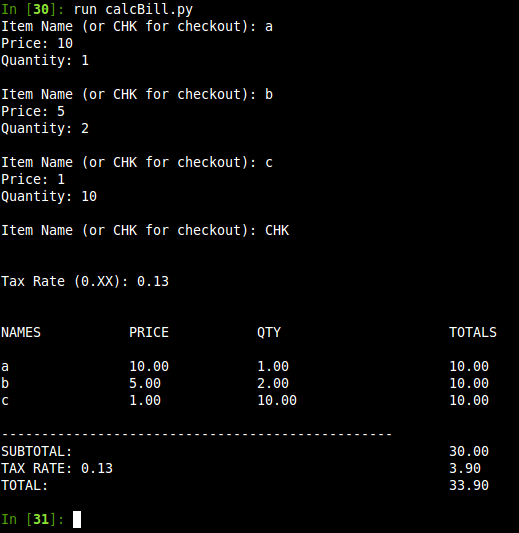
4. Cipher¶
- Write a function to encript a string by exchanging letters with a letter some fixed number of positions down the alphabet. The below example shows what the alphabet would be encripted to with an offset of 3. This function must take a string and an offset.
abcdefghijklmnopqrstuvwxyz
defghijklmnopqrstuvwxyzabc
WARNING. THIS IS NOT JUST A STRING ROTATION
So with the cipher, if we had the string “hello world”, it would turn into “khoor zruog”.
- Write a function to de-ecript a string by doing the opposite of the first function, effectively undoing the encryption. This function must take a string and an offset as parameters.
NOTES:
Feel free to create helper functions
- It is possible to write 1 function to do both, however, you don’t have to
- If you do, explain this with comments
You can assume only lowercase letters
You can assume an offset between (0-26)
5. Basebal Stats¶
Download the two ‘.csv’ files representing the player statistics for the American League the National League .
Write a program which will plot At Bats (AB) vs. On Base Percentage (OBP) for all players in both leagues who had more than 100 at bats. Additionally, the radius of each data point in the graph should be equal to that player’s Home Run (HR) count. Lastly, the data points should be coloured based on the league; all American League data points should be blue and all National League data points should be red.
Make sure that your axis are also labeled properly with a title matching the figure below.
NOTES:
- MLB is weird, their percentages are not actually percentages, they’re decimal values. So just plot yours like the figure below with the percentages as decimal values
- What’s cool about this plot is that 4 dimensions are being represented: AB, OBP, HR, and League!
- Size of plot does not matter (like if yours is short and fat vs. square).
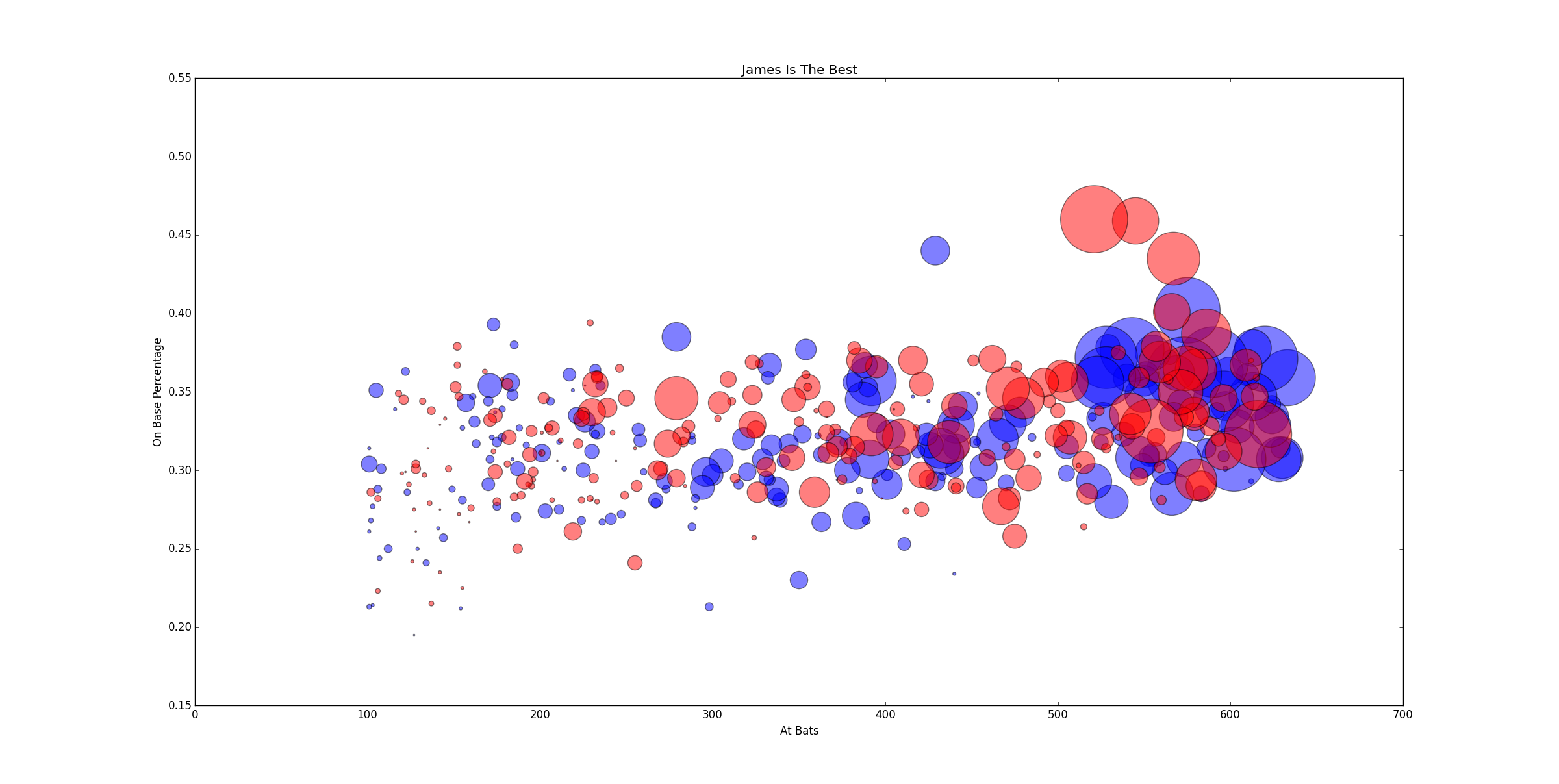
6. Palindromes semordnilaP¶
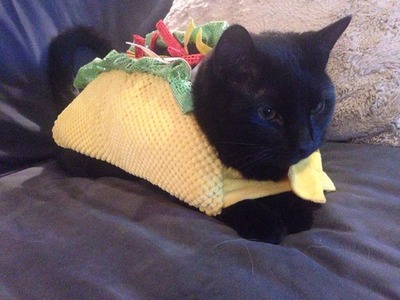
Download this small file of palindromes.
Write a program to check if a string is a palindrome. Your program should read the file one line at a time and check if it is a palindrome. Your program should also ignore case, ignore spaces, and ignore any punctuation at the end of the strings. See the below example.
NOTES:
- Must print out the original string
- Must work for an arbitrary file
- Must eliminate spaces
- Must eliminate punctuation at the end of the strings
- Must ignore the case (upper case vs. lower case)
- The strings must be printed out with quotations like in the below example
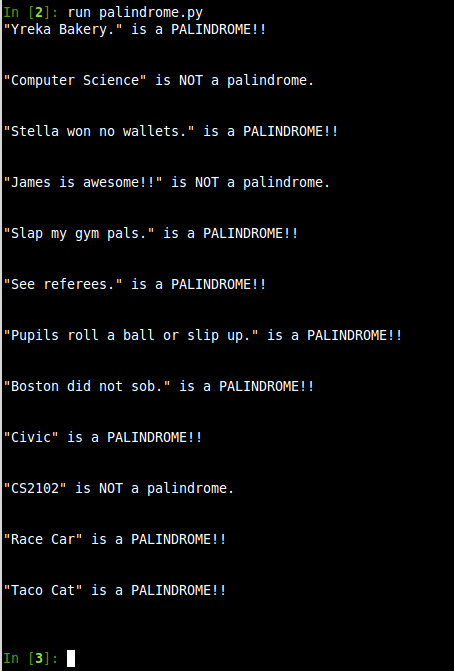
7. Rock some Stocks (DO NOT EVEN PREP FOR THIS ONE ANYMORE)¶
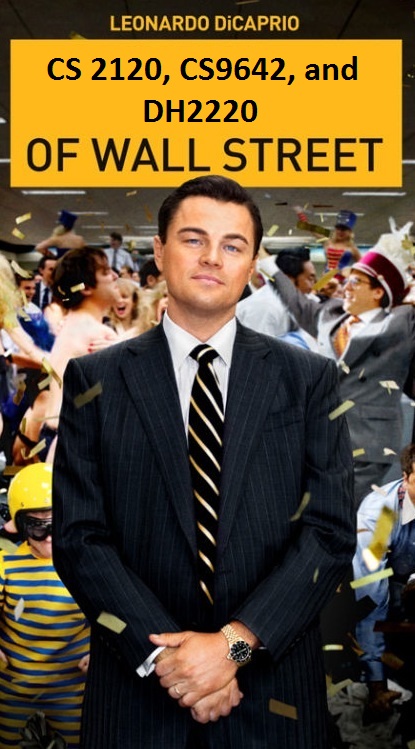
Do not bother prepping this one. Yahoo recently, like 2 weeks ago, took away their stock website thing
Take this code and use it to write a program to:
OR DON’T!
Pull stock information from the internet
- Plot the opening, high, and lows over time
- Opening is a blue dashed line
- Highs are red squares
- Lows are green triangles
DON’T DO THIS ONE
See the below example for how my plot looked when I used the ticker BNS.
NOTES:
- YOU REALLY SHOULD IGNORE THIS ONE
- You WILL need to alter the
output_path
variable. - I stole the code from (http://stackoverflow.com/questions/12433076/download-history-stock-prices-automatically-from-yahoo-finance-in-python)
- When adding the labels to the x-axis, I suggest not showing every single date (unreadable). I did every 100th date, but pick whatever you want
- Don’t worry too much if the last date on the x-axis does not align with the last data point
- Mind the direction of the dates (time ->)
- FOR REAL, SKIP THIS... IT WON’T WORK
- Size of plot does not matter (like if yours is short and fat vs. square).
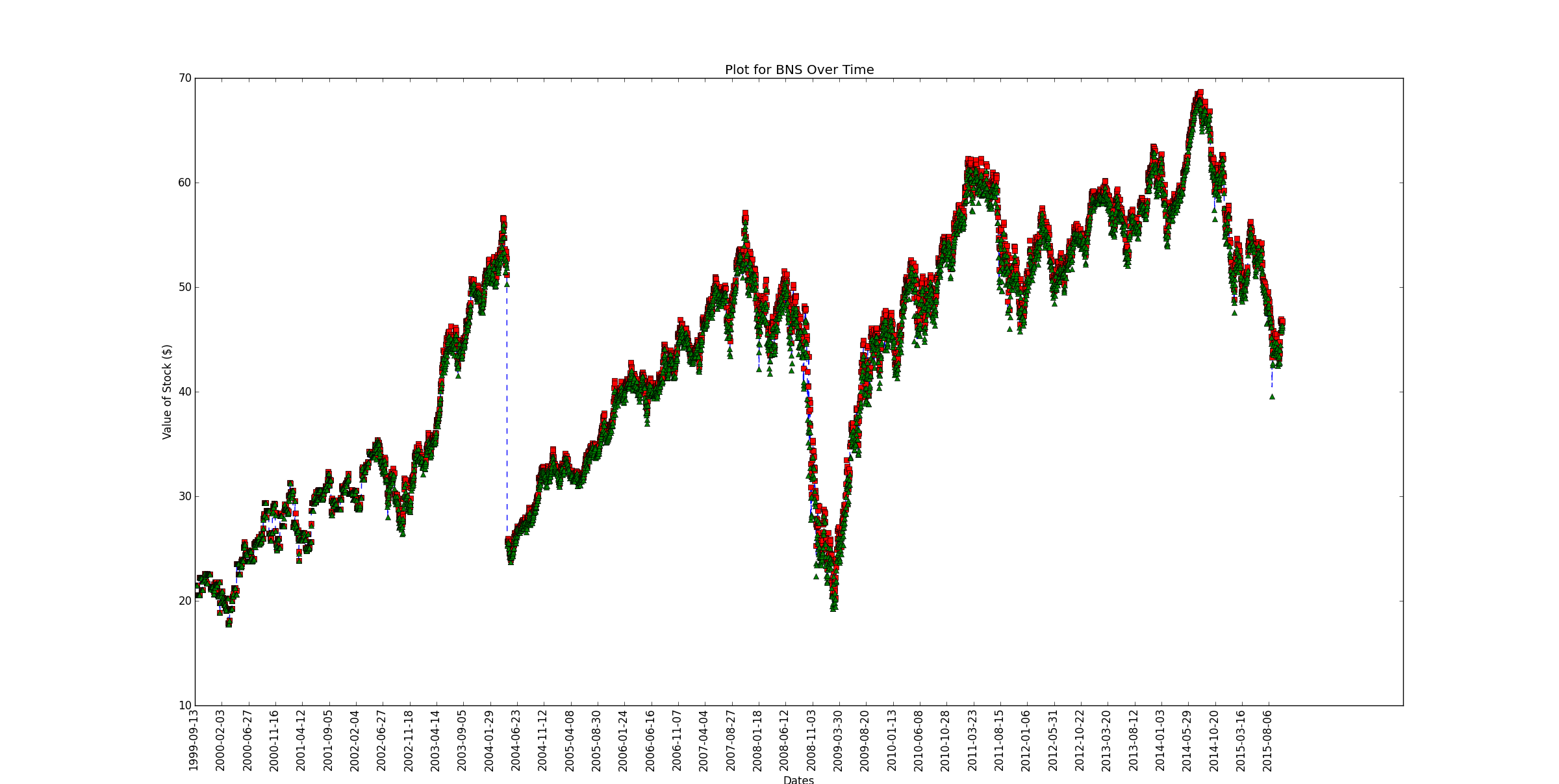
8. Fibonacci¶
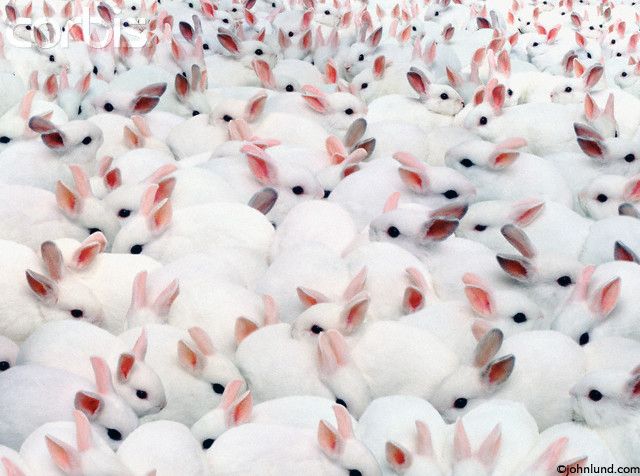
The Fibonacci numbers are the numbers in the following sequence:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, ...
Basically:
With the exception of the first two numbers, each number is the sum of its two preceding numbers, so...
0 + 1 = 1
1 + 1 = 2
1 + 2 = 3
2 +3 = 5
and the number to come after 144 would be 89 + 144 (which is 233)
The catch is that we must have the first two numbers already given to us. We’ll use the zeroth as 0 and the first as 1.
So we say:
F(0) = 0
F(1) = 1
and the nth Fibonacci number is:
F(n) = F(n-1) + F(n-2)
- Write me a recursive function to compute the nth Fibonacci number.
- Write me a non-recursive function to compute the nth Fibonacci number.
call your method fib(n).