Assignment #1: Starbucks Density in North America¶
- Worth: 10%
- DUE: October 5th at 11:55pm; submitted on OWL.
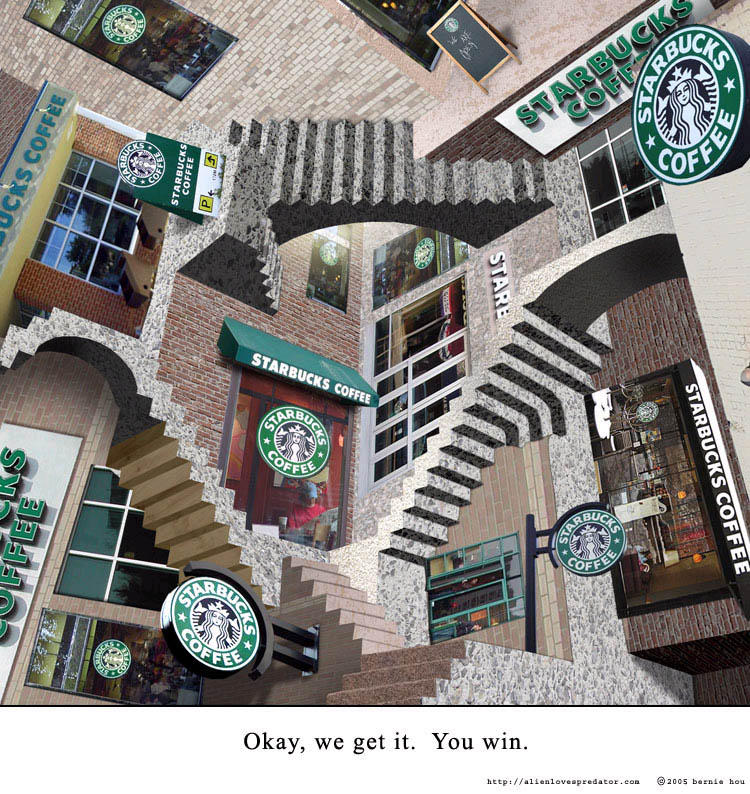
In this assignment, you will use a real dataset containing the locations of all North American Starbuck’s Coffee houses (as of a few years ago) to compute the density of Starbucks on a given patch of the Earth’s surface.
You will need the data file starbucks.csv . Download this to your computer and save it in the same directory that you will save your assignment.
To make life easier for the first assignment, you don’t have to start from scratch. I’ve already started a file for you to use as a template. This is also somewhat realistic for a scientific programmer; you don’t often start completely from scratch... usually, you’re trying to modify someone else’s code that you downloaded or inherited.
Download asn1.py to get you started.
The steps you need to do are laid out, in detail (and suggested order) below.
Complete Latitude/Longitude Conversion Function¶
Humans like to express latitudes and longitudes in degrees, minutes and seconds. You could work with those units in Python, but your computations (and, hence, code) will be much cleaner if you convert to the single unit “degrees”, using fractions of a degree to represent arcminutes and arcseconds (don’t worry, I swear you that this isn’t that complicated).
Your first task will be to fill out the code for the function convert_to_decimal()
.
The parameters are a latitude (or longitude) in degrees, arcminutes and arcseonds. The
function should return the same latitude (or longitude) as a single in decimal degrees
(a single value of type float
). If you’re not sure how to compute the conversion,
do some reading.
Have a peek at the data-loading function¶
This time, the function that loads the data is a freebie. It contains some stuff we haven’t discussed in class yet, but it’s pretty easy to figure out what’s going on if you look at it. So look at it. Get used to looking at code that isn’t yours, and may use unfamiliar ideas/idioms/patterns, and trying to figure out what it does. This isn’t always easy (sometimes it’s very hard), but you’ll spend a lot of time doing it (whether you want to or not!).
This data in the .csv file is fortunately already in decimal format so you will not need to convert it with convert_to_decimal()
, however, you still need to do part 1 and make sure it works properly even though you might not actually use convert_to_decimal()
for the below parts!!!!!!
Complete the function to compute lat-long rectangle area¶
We want to compute the density of Starbucks, not just the raw number of them.
A reasonable measure for this is ‘Starbucks per square kilometre’. To compute this,
we first need to know how to find the area of a lat-long rectangle. The function subtended_area()
takes as parameters the ‘bottom left’ and ‘top right’ corners of a lat-long rectangle.
It should return the area of that lat-long rectangle in square kilometers.
To save you some solid angle computations: given two lat/long pairs, the formula to compute the subtended area is:

where R is the radius of the Earth (6378.1 km).
Warning
Do Python’s trig functions (e.g. math.sin
or numpy.sin
) expect parameters in degrees or radians? Be careful!
Complete the function that counts the number of Starbucks in a region¶
The main event. Counting up the number of Starbucks in a given area. The function
num_starbucks()
is already set up to loop over every Starbucks location in a list.
All you have to do is remember to pass in the list. Have a look a the loop. Even if we haven’t
formally discussed this structure in class yet, it should be pretty clear what it’s doing.
Your work is in the body of the loop. Each time through the loop we’ll be considering a new
Starbucks location. The existing code will put the current location’s latitude in loc_lat
and
longitude in loc_lon
. Your job is to figure out if this location falls in the area between
the lat/long pairs defining your region. Specifically, if lat1 < loc_lat < lat2
and lon1 < loc_lon < lon2
,
then the current Starbucks is in the region. Otherwise, it isn’t.
You need to keep track of how many Starbucks there are in the region and then return that value.
Finally¶
Finally, you can fill in a convenience function starbucks_per_kmsq()
which, given
subtending lat/long pairs, returns the density of Starbucks.
- Use
load_asn1_data()
to load the locations and store them in a variable. - Compute the number of Starbucks in the region
- Compute the size (area) of the region
- Divide number of Starbucks by area to get density
- return density
Now try it!¶
Try your code. Try small regions and big regions. What area has the highest Starbucks density you can find? The lowest?
What to submit¶
Your version of
asn1.py
Make sure your NAME and STUDENT NUMBER appear in a comment at the top of the program.
List anyone you worked with in the comments, too
- A text file describing the areas you found with the highest, and lowest, Starbucks densities and a short description of how you found them.
- Don’t worry about finding the HIGHEST or LOWEST density, just try a few and pick your highest and lowest.
Some hints¶
Work on one function at a time.
Get each function working perfectly before you go on to the next one.
- Test each function as you write it.
- This is a really nice thing about Python: you can call your function right from the interpreter prompt and see what result gets returned. Does it look reasonable? Good! Check it!
If you need help, ask! Drop by my office. Ask the TA during lab. We’re here to help.
Remember that it is not only ok but actively encouraged to work together.
FAQ:¶
- Does my text file have enough details?
- Probably. The shorter the better.
- I don’t know how to do X.
- OK, go to google.ca and type in X.
- My thing keeps telling me ERROR: File `u’SOMETHING’` not found.
- Then the file isn’t where python is looking.
- But density will grow larger the smaller I make the area (aren’t I so smart).
- Congratulations, you understand basic math.
- Is my area a high/low enough density?
- I really don’t care how high/low it is. Just try a few things and see what you get.
- But I never used the one function!!!!1!
- Fine, but write the code anyways and make sure it works.
- But the degrees values don’t specify a cardinal direction!
- Make use of changing +/- if you need to change hemispheres.
- Wtf do the functions do that you gave me?
- Read the descriptions.
- Some of the code in the functions you gave us look like magic.
- That’s because it’s magic.
- Do I have enough comments?
- I don’t know, maybe? If you’re looking at code and have to ask if you should comment it... just comment it. That said, don’t write me a book.