CS212: Introduction to Software Engineering Project Description Fall 2008
Version 1.0 May 2008
1. Project Overview
The software
to be developed is a bowling score keeping system and tournament system which will calculate the score of an individual based on the pins they knock down, keep track of teams and keep track of teams bowling in a tournament. An administrative user will enter in the team members names, and the teams initial rankings. The administrator will also enter the names of groups who just come to play but are not involved in the tournament.
2. Explanation of
Terms
- 5 Pin Bowling: The game of bowling where each bowler has 5 pins to knock down and each bowler gets 3 balls for each turn (frame)
- 10 Pin Bowling: The game of bowling where each bowler has 10 pins to knock down and each bowler gets 2 balls for each turn (frame)
- Strike: In both 5 pin and 10 pin bowling, a strike occurs when the bowler knocks down all the pins on his/her turn with his/her first ball.
- Spare: In both 5 pin and 10 pin bowling, a spare occurs when the bowler knocks down all the pins on his/her turn using only his/her first ball and his/her second ball.
- Turkey: In both 5 pin and 10 pin bowling, a turkey ccurs when the bowler gets 3 consecutive strikes.
- Random Pin Selection Mode: For our system, this will occur, when the system randoming picks which pins to knock down in order to simulate a game, rather than allowing the bowler to enter which pins were knocked down.
3. Non-Functional
Requirements
(Non-functional
requirements are requirements which do not have to do with the specific
input-output behaviour of the system)
- The system must run on the Computer Science department’s GAUL Unix system
- The system must be developed in Java
- The system must have a graphical user interface
- The system must not use Netscape, Explorer, Lynx or
any other Web browser, due to the impossibility of authorizing such things
as CGI scripts on the GAUL system Web server
- Quality:
- User Friendliness
- The system must be easy to use. For example, a user
must be able to navigate easily between menus and must have the option of
quitting or going back to a previous menu at all times
- Messages as well as errors must be reported
correctly and consistently
- Reusability
- You must try, as much as possible, to make your
code reusable
- You must make sure that your code is commented (at
least a description of each class and each method must be given) so that
it is can be modified by others.
4. Functional
Requirements
(Functional requirements are
requirements on the input-output behaviour of the system)
a) System Startup and Exit
- The system must have the executable name of UWOBowl
- The system
must start with a screen which allows the user to go into either game mode
or tournament mode. See explanations of both modes below.
- If the –admin option is used on the command line, the system must start up in administrative
user mode (thus the command java UWOBowl –admin would start it in administrative
mode)
- The user must be able to quit at any time in any mode, terminating the
program.
b) Game Mode:
In game mode, the user is about to play a game with up to 3 other players. The system allow the user to enter the 4 players names and then must keep track of which pins were knocked down and calculate the scores for each player. The system must allow for five pin bowling and ten pin bowling.
- Starting a new game:
- Some players will be in the league and their names and user ids will already be in the system. The user must be able to pick one of the league names as the player for this game OR pick a team and then the team names will be who plays in this game OR enter his/her name (see b.1.2 below)
- Some of the players will NOT be in the league, thus their names will not be in the system. Their first name must be entered and their last name may be entered
- The system must allow 1 to 4 players to be entered.
- In real bowling there are always 10 frames but for testing purposes we will all the user to pick between a 4 frame game and a 10 frame game.
- Also, for testing purposes we will allow the user to select a random pin selection that randomly decides how many of the pins to knock down. If the user does NOT select the random pin selection, then the user must be able to indicate for each frame for each player which pins were knocked down.
- Finally, the user must be able to select if the game will be a 10 pin game or a 5 pin game.
- Then to start the game the system must display the first names and/or last names of the players playing the game and at least three of the first 4 or 10 frames. It should look something like this:
Player |
Frame 1 |
Frame 2 |
Frame 3 |
Homer Simpson |
|
|
|
Bart Simpson |
|
|
|
Marge Simpson |
|
|
|
Lisa |
|
|
|
-
Playing the game:
- If the player is playing the random pin selection, then have some way for the first player to throw his ball and knock down the pins. Your program should indicate which pins were knocked down. If the game is 10 pin then 0 to 10 of the pins should be knocked down with the first ball. If the user is playing 5 pin, then 0-5 of the pins would have been knocked down. Each player should be able to throw two balls for 10 pin OR three balls for 5 pin. After each ball calculate and display that players score. After the last ball has been thrown you should indicate that it is the next player's turn. If the player is playing where he/she indicates which pins were hit, then your system should somehow allow the user to pick the pins that were knocked down for each ball thrown.
- For each players turn, you must indicate:
- Which pins are still standing
- if the player knocks down all the pins on the first ball then display "STRIKE" somewhere
- If the player knocks down all the pins on his/her second ball, then display "SPARE" somewhere
- If the player has a shot where all he/she has to knock down the 2 outer pins in the back row, then indicate "SPLIT --> HARD" somehow.
- Turkey (if some gets 3 or more strikes in a row)
- For the final frame you must indicate which player won the game.
- Scoring:
- 5-Pin
- Each player gets to throw 3 balls per frame
- The pins are worth different values. The values are indicated in the following image:
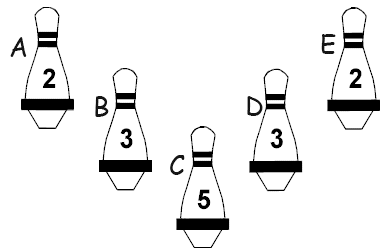
- 3 BALLS PLAYED: Add up the value of the pins knocked over to get the bowlers score for that frame. For example, from the image above, if a player knocked down on his/her first ball pins B and C, his/her score so far would be 8, then if the player knocked down pin A on the second ball, his/her score would be 10 and then if the player didn't knock down any pins on his/her last ball, the final score for that frame for that player would be 10. Accumulate the total score by adding the frame score on to the previous total score..
- SPARE. If the player, say Sue, knocked down all the pins with the first 2 balls, then she bowled a spare and the turn goes to the next player and no value is put in for that frame for Sue, instead her score for that frame would have a diagonal slash in it. Then, on the next frame, when it is Sue's first ball again, whatever Sue bowls on the FIRST ball gets added to 15 and that is Sues score for the previous frame, thus the slash is replaced by the new score.Then Sue also gets the value of the first ball on this frame also.
- STRIKE. If the player, say Fred, knocked down all the pins with his first ball, then he bowled a strike and the turn goes to the next player and no value is put in for that frame for Fred, instead her score for that frame would have an X in it. Then, on the next frame, when it is Fred's first ball again, whatever Fred bowls on the FIRST and SECOND balls gets added to 15 and that is Fred's score for the previous frame, thus the X is replaced by the new score.Then Fred also gets the value of the first two balls on this frame also. If Fred bowled a second strike, then you still cannot calculate Fred's score for the first strike, because you must add his 2 balls and with 2 strikes he would only have one, so you would have to wait for the next frame to calculate his score.If a player gets a strike in the second last frame or the last frame, then he/she could get one or two extra balls to bowl.
- Click here for more on the scoring of 5-pin bowling
- 10-Pin
- Each player gets to throw 2 balls per frame
- All the pins are worth the same amount, 1 point each.
- The pin layout is as follows:
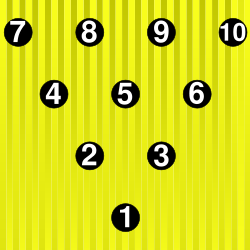
- 2 BALLS, NOT ALL BALLS KNOCKED DOWN --> Add up the total of all the pins that were knocked down. This is the score for this frame, add it to the total of the previous frames.
- SPARE: Same scoring as for 5 pin
- STRIKE: Same scoring as for 5 pin
- Click here for more scoring information on 10 pin bowling
- League Players Scores:
- For people just playing for fun (non-league bowlers), you do not need to save the players score but for players who are in the league, you need to save the time and date and scores and whether or not it was 5 pin or 10 pin and whether or not it was a 4 frame game or a 10 frame game for every game they play. If a league player quits in the middle of a game (without completing it), DO NOT save any information about the game.
- If this was a team from the league playing this game, you must save the team's score and the date and time this game was played.
- Quitting
- At any time,
the user must be able to quit from game mode
c) Administrator Mode:
- Entering and Removing League players:
- The admin user must be able to add league players. Each league player must have a first name(1 to 20 letters)and a last name (1 to 20 letters)and a unique id. The unique id must be 4 characters long and contain only letters (non-case sensitive) and numbers. The unique id must be entered by the administrative user and not generated by the system.
- Each league player will have a series of scores associated with him/her that contain the date and time and score of every game played by the league player.
- The administrative user may delete a league player at any time. If a league player is associated with one or more teams then he/she is also dropped from the team. If he/she is the only member of a team, then the team should be deleted also.
- List all the league players and allow the admin user to select on a player to get info about the player such as: first name, last name, user id, which teams he/she is on, which games he/she has played in and information about those games
-
Adding and Removing Teams:
- The admin user can add a team of league players. Each team of league players must have a unique name. The name must be 1 to 30 letters and/or spaces. The team name must start with a letter and consist of only letters or spaces Each team must also be given a ranking between 1 and 5. Teams with a ranking of 1 are the best, teams with a ranking of 5 are the worst..
- Each team can have up to 4 players on it and must have at least one player on it. The players must each be a league players. Do not enter the same player more than once on one teachm, however league players can be on more than one team.
- Each team can be deleted by the administrative user. If a team is deleted, the players on it still exist.
- List all the teams and allow the admin user to select on a team to get info such as: team name, team ranking, players on the team, which tournaments the team has played in in the past, and which tournaments the team is currently participating in.
- Setting up a tournament:
- To set up a tournament, the admin user must decide which teams will be in the tournament and then which teams will play against which other team either: by ranking or randomly assign the teams for the initial round. Two teams will go against each other in every round, unless there is an odd number of teams in a round, then, if the admin user is using the ranking tournament, the team with the highest rank will automatically move to the next round. If the admin user is doing it randomly then, with an odd number of teams, a random team will automatically advance to the next round.
- The most number of teams that can participate in a tournament is 8, the least that can participate is 2 teams
- There can be more than one tournament happening at one time so the admin user must be able to add new tournaments and delete tournaments
- A tournament must have a unique name that is no longer than 30 letters and/or spaces and the name must have at least 1 letter.
- The same team may play in several different tournaments
- The admin user must be able to indicate if this is 10-pin tournament or a 5 pin tournament
- Once the admin user has picked the initial teams for the first round of a tournament and the first round schedule has been generated, the teams participating may NOT change, they must remain fixed until the end of the tournament. A team or a league player can NOT be deleted while it is participating in a current tournament, however once the tournament is completed then the team or player can be deleted but the required tournament information must be retained for viewing purposes. (see d.3)
- The admin user is only responsible for setting up the initial schedule for the tournament, after that, a user goes into tournament mode to enter the scores and figure out which teams move up.
- Assume we want to set up a schedule with the following teams: Team A, Team Alpha, Team B, Team C, The Big Bowlers, Western Bowlers and Comp Sci Nerds. Assume we are doing the random assigning of teams playing each other, thus the initial schedule that the admin user might generate would look like this:
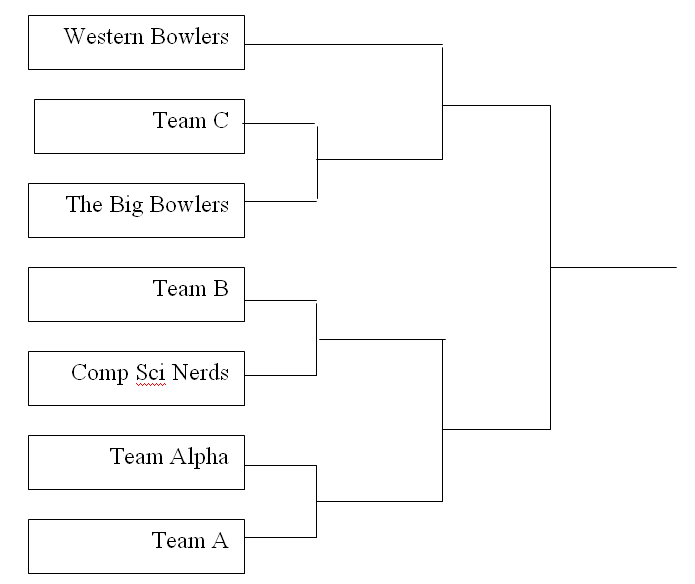
- Once the tournament has been set up initially, the user must go back into tournament mode in order to enter the bowling scores to see which team moves up.
- An admin user can delete current tournaments or previous tournaments (tournaments that have been finished)
- List all the tournaments, past and currently running, and allow the admin user to see the name of each tournament and select a tournament to display the layout.
d) Tournament Mode
-
General
- A user in the tournament mode must be able to pick a tournament from the list of tournaments available.
- Then the user must be able to enter one or more teams scores. All the scores for each round must be entered before a user can move to the next round.
- Once all the scores for all the teams on a round have been entered, the second round of play is generated. If a ranking system is being used for the tournaments and if you have an odd of number of teams then the team with the highest rank will automatically get a buy into the next round. If the random system is being used, then the system will randomly pick a team to move into the next round automatically in the case of an odd number of teams in a round.
- If the user quits the system, the current status of the tournament must be saved. Thus all scores entered so far must be saved.
-
Entering Scores
- There are two ways to enter the scores for a team in a round of a tournament:
- The user could just enter the final score for each member on the team.
- The user could pick from one of the games that a team has participated in and have that value entered in as the team score
- NOTE: if the game the player played in was a 4 frame game, then calculate what his score would have been if he was bowling 10 frames, i.e. take his average over the 4 frames and multiply that by 10
- Only 10 pins game scores can be used in 10 pin tournaments and only 5 pin game scores can be used in 5 pin tournaments
- If a team has less than 4 players, than the average value of the number of team members that do exist will be entered as the score for the other players.
- After the scores from two teams competing against each other is entered, then the team with the higher total score should move to the next round.
- Viewing Current and Previous Tournament and Team and Player Data
- An tournament user
must be able to view the following things;
- The layout of a current tournament (showing which teams have moved on to the next round and total score for each teams)
- All the rounds for previous tournaments, showing the teams who played and winners of each round, the final winner and total score for each team. You do NOT need to show which players were on the team at the time of a PREVIOUS tournament.
- The top 3 teams in the league, based on the highest game scores of all games of all the team currently in the system (do not include deleted teams) in 5 pin and the top 3 in 10 pin. Average 4 frame games over 10 frames
- The top 3 players in the league, based on the highest game scores of all games of all the players currently in the system (do not include deleted players) in 5 pin and the top 3 in 10 pin. Average 4 frame games over 10 frames.
- List all the teams and allow the tournament user to select on a team to get info such as: team name, team ranking, players on the team, which tournaments the team has played in in the past, and which tournaments the team is currently participating in.
- If the user chooses so, then show the total scores and dates and time for each game the team played in. If the team had fewer than 4 players, do include the average for the missing players in the display (not just the total of the players who did play in the game)
- List all the league players and allow the tournament user to select on a player to get info about the player such as: first name, last name, user id, which teams he/she is on, which tournaments he/she is CURRENTLY playing in (you do not need to indicate which tournaments he/she has played on in the past).
- If the user chooses so, then show the games scores, dates and times and type of game (5 pin or 10 pin) and number of frames for the selected player.
- Printing out a tournament
-
A tournament user must be able to print out the layout of a tournament either so far (if it is still going on) or all rounds (if it has completed). To do this just put the data in a nice format in an HTML file.
e) Display
All three types of users: game, tournament and admin must be able to choose between three
skins. A skin is a look and feel and/or a theme for the interface. For
example all the buttons/text/menus would have the same colour scheme in a
particular skin. 3% of your final mark for acceptance testing will be
based on the aesthetic appeal/creativity/originality of your skins. HINT:
Java swing has a look and feel class to help. If you want to do some cool
stuff, do a search on Java, "Look and Feel" for sample code. BUT
LEAVE THIS TO THE END, make sure all the other stuff works first :-)
5. Revision History
Revision
history of this document, in reverse order of versions released:
- Version 1.0, May 30, 2008. Internal release to
instructor
- Version 1.1, Sept 22, 2008, Changes to specs as required by student questions
- Version 1.2., Sept 29, 2008, Changes to specs to help with display
- Version 1.3, Oct 9, 2008, Changes due to issues with how long to store the data.